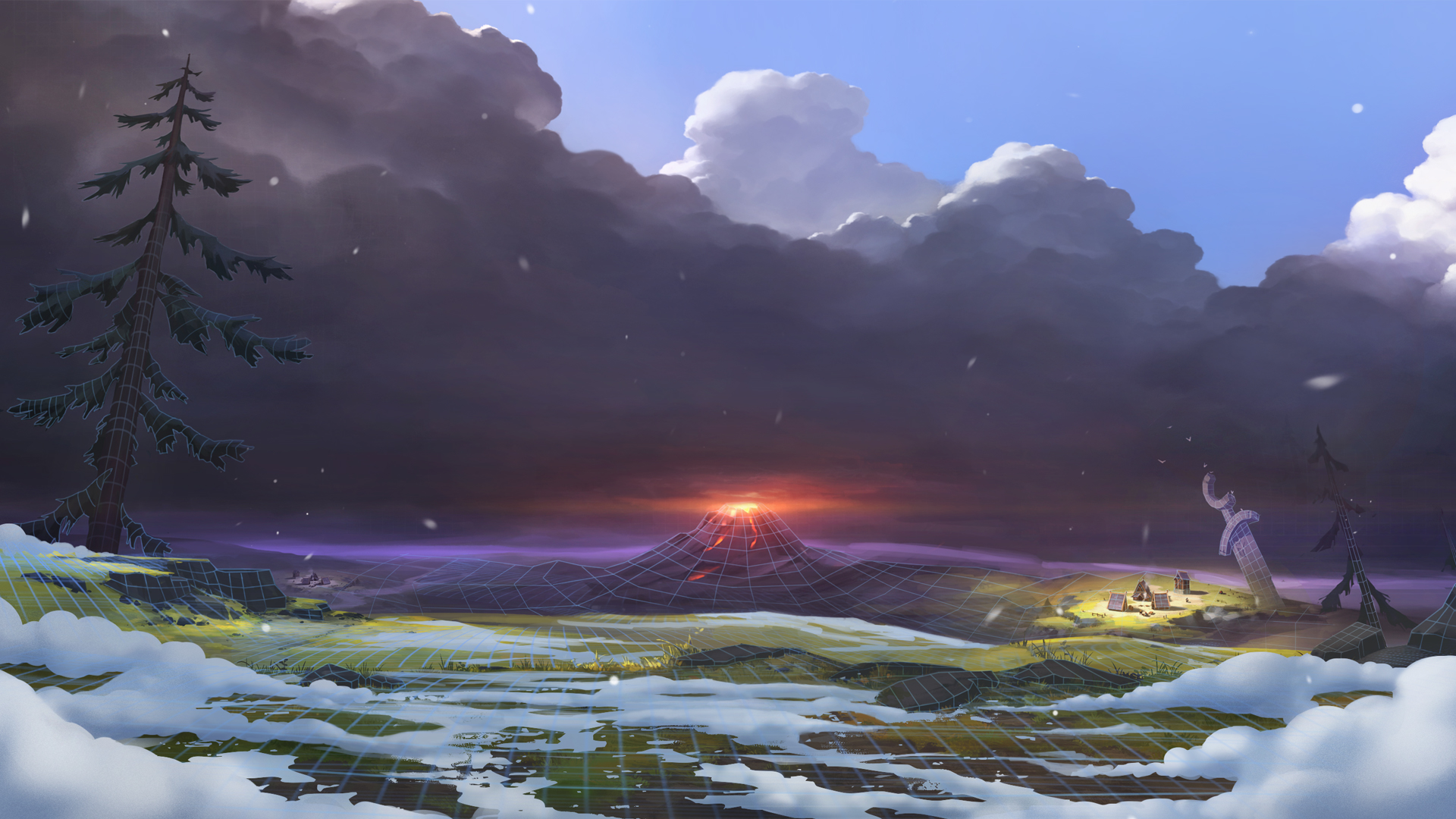
LOCAL FILES
Please ensure your mod is stored in the folder “mods”, located in the root folder of Northgard local files. To open Northgard local files, right click on Northgard in Steam > Properties > Local files > Browse local files.
Each folder in “Northgard/mods” corresponds to a local mod, with organized files as below:
map.dat: an edited map.
script.hx: a script, which must be associated to an edited map; a script cannot exist without.
cdb.diff: the file that modifies all allowed game data.
The folder name is used for your mod name as well.
CREATE AN EDITED MAP
To create a new map, click on « Create Map » located in this menu.
As you save your map, a folder containing all your mod local files will automatically be generated, as stated above.
CREATE A SCRIPT
Getting started
A script in Northgard is a text file written in Haxe which will be read and interpreted by the game during gameplay. To add a script to your map, simply create a new text file named “script.hx” in your mod folder.
Your script will be an empty file at this point. To obtain some visible some behaviour, you will need to add the following to your file :
function init() { } function regularUpdate(dt : Float) { }
The init() function is called each time at the execution of your script. It can be the first time you start a game with your mod, or when you load an ongoing saved game.
If you need to execute a piece of code only at the start of a new game, you can test for state.time to check the passed time. A time of 0 is when the game starts for the first time.
function init() { if(state.time == 0){ // This part will be executed on the first launch only. } // This part is executed each time the script is loaded. }
function regularUpdate(dt : Float) { if(state.time >= 10){ me().triggerVictory(VictoryKind.VFame); // After 10 seconds, we instantly grant a victory by Fame to the main player. } }
Variables and data persistency
var myInt = 10; // global variable of type Int initialized at 10 var myFloat = 3.8; // global variable of type float initialized at 3.8 var myZone; // global variable of unknown type
function init() { var myLocalInt = 20; // local variable of type int initialized at 20 myFloat = 1.; // We change the value of myFloat to 1; myZone= getZone(43); // We use the variable of unknown type to save a zone } // Past this point, myLocalInt doesn’t exist anymore
myFloat = 3.8; // global variable I want to be persistent var myZone; // variable with a “var” prefix won’t be restored
function saveState() { state.scriptProps = { myFloat : myFloat // Your variable to save. Ensure the name of the variable is repeated twice here } }
In saveState(), we assign a structure to state.scriptProps. This allows the game to include this structure and its content to the save data.
Be sure to repeat the variable name twice in the structure, else the data won’t be properly loaded in the variable when executing the script. Also, the variables you want to save shouldn’t be prefixed by a var. This is a technical specification for the game to properly locate your variable and restore its value.
Blocking functions and synchronization
function init() { var zone = getZone(182); // get a zone where we added a warrior var warrior = pz.getUnit(Unit.Warrior); // get the warrior moveCamera({x:560, y:612}, 0.8); // move the camera at a certain speed toward coordinates [560, 612] moveUnit(warrior, 10, 0, true); // Move warrior by 10 units slowly walking } // Past this point, myLocalInt doesn’t exist anymore
@async moveCamera({x:560, y:612}, 0.8); // dont wait for this function to end moveUnit(rig, 10, 0, true);
// Or
@async { // bypass all blocking functions in the block moveCamera({x:560, y:612}, 0.8); moveUnit(rig, 10, 0, true); }
function init(){ var lookAtZone = getZone(80); @sync { for(zone in state.zones) { for(u in zone.units) u.orientToTarget(lookAtZone); } } }
function init(){ var zone = getZone(152); var rig = zone.getUnit(Unit.Hero09); var brand = zone.getUnit(Unit.Hero07); var svarn = zone.getUnit(Unit.Hero08);
@split[ moveUnit(rig, 10, 0, true), // rig move slowly walking { wait(1.); moveUnit(svarn, 10, 0, false); // svarn is late and hurry to join his friends }, { wait(0.5); moveUnit(brand, 10, 0, true); // brand walk after waiting a bit } ]; moveCamera(554, 257, 0.08); // The camera will pan once svarn start moving }
Northgard Editor
To edit your scripts, you can use any text editor, but to benefit from features such as the syntaxic coloration and error check, you are provided with Northgard Editor, a subset of the tool used to develop Northgard. You can find it in your Northgard install folder.
To edit your script with Northgard Editor, start “Editor.exe” and drag and drop your script file in the editor window. It will check everything you are writing. If your code contains invalid syntax or unauthorized functions, the editor will highlight it in red and will display an error message.
Don’t forget to save your changes with the “Ctrl + S” shortcut key once done !
For more information
You can find a script file “script.hx” summarizing the above in the folder Northgard/NGEditor/res. You can simply past it in your mod folder and modify it with Northgard Editor to your suit needs.
You’ll find the complete online script documentation by clicking on “Documentation” in Northgard Editor or by clicking on this link.
If you’d rather go for our local version, open Northgard/NGEditor/documentation/index.html in your preferred browser.
SCRIPTING FOR MULTIPLAYER
You can find a detailed explanation on how to script for multiplayer Multiplayer Script by following this link.
MODIFY YOUR GAME DATA
Open Northgard Editor. To create a .diff file and modify your mod data, click on Database > Diff > Create. Save the file in your mod folder under the name “cdb.diff”. Once your file is created, click on Database > Diff > Load to edit it.
When a .diff file is loaded in Northgard Editor, any editable field you change will be added to the file. Be cautious, as an auto save system is triggered for each change you make. Press Ctrl + Z to cancel your last action.
TEST YOUR MOD
Satisfied with your mod? You need to test it before releasing it on Steam Workshop. In the Single “New Game” menu, select your local mod and start a game. You can also come back to your game later by saving and loading it as you would normally.
Once you tested and verified your mod function properly, upload it on Steam Workshop using the “Upload Mod” menu.
MOD CUSTOMIZATION
To add a mod preview image in the Steam Workshop, add a file named « preview.jpg » in your local mod folder. This preview will sync next time you upload your mod on the worskhop
OPEN NORTHGARD EDITOR
You will find the Northgard Editor here: Northgard/NGEditor/Editor.exe
Right now, the Northgard Editor tool is only available on PC.
CUSTOM TRANSLATION
Create your translation
You can now make your own translations of the game. For that, you’ll need to retrieve the english translation files at the following links :
(Right click and download)
Once you have downloaded the files, follow these steps to create your translation :
Create a “langs” folder into your Northgard game folder.
Create a folder into langs with the name you want to give your translation.
Save texts.xml and export.xml into your new translation folder.
Edit texts.xml and export.xml to replace the english sentences with the translation you want, then save them.
Now that your translation is ready, simply start Northgard and go to the ingame options. In the language selection box, you will see your folder name at the end of the list. Select it and ensure the texts are translated as you intended.
Plural and lang specifics
Some languages have entirely different words between singular and plural. For such, each item name in the export.xml can be associated with a specific name for plural, nominative, dative and such with <langs.xxx> markup.
Below, the english version.
<Food>
<name>Food</name>
<desc>[Food] is necessary to feed your clan. Stock up on food before winter. Avoid starvation at all costs.</desc>
<langs.plural>Food</langs.plural> // The plural name for Food. This will ommit the ‘s’ which is automatically added otherwise.
</Food>
And the russian version.
<Food> <name>Еда</name> <langs.plural>Еда</langs.plural> <langs.genitive>еды</langs.genitive> <langs.dative>еде</langs.dative> <langs.accusative>еду</langs.accusative> <langs.nominativeLc>еда</langs.nominativeLc> <desc>[Food] необходима, чтобы прокормить клан. Запасайте еду на зиму и избегайте голода любой ценой.</desc> <langs.plural>Еда</langs.plural> </Food>
Install a translation
To install a translation, simply download the translation folder you wish to use and save it under northgard/langs/. If the translation works properly, you will see it at the end of the Language selection list. (See above.)
NORTHGARD EDITOR F.A.Q
“Resources” tab only shows a “No longer exists” notification.
Click on Project > Open and select the NGEditor folder contained in your game local files.
I’m trying to open a .diff file but nothing happens.
Click on Database > View. It should be displayed now.
I’ve just updated a mod on the Steam Workshop, I’ve changed the Mod kind but I still see the “old version” in the upload menu.
In case you have subscribed to your own mod, a self-resolving conflict may occur between the Steam Workshop information and your outdated downloaded mod.